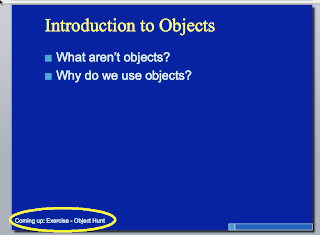
tell application "Microsoft PowerPoint"
set oPres to active presentation
set mySlide to 1
tell active presentation to set currentSlide to slide mySlide
set titleTypes to {placeholder type title placeholder, placeholder type center title placeholder, placeholder type vertical title placeholder}
set footerTypes to {placeholder type footer placeholder}
repeat with currentSlide in (get every slide of oPres)
if mySlide > 1 then
-- Get the title of the current slide
repeat with i from 1 to count shapes of currentSlide
set currentShape to shape i of currentSlide
set aType to placeholder type of currentShape
if aType is not missing value and aType is in titleTypes then set myTitle to currentShape
end repeat
-- Get the footer of the previous slide
repeat with i from 1 to count shapes of prevSlide
set currentShape to shape i of prevSlide
set aType to placeholder type of currentShape
if aType is in footerTypes then set myFooter to currentShape
end repeat
-- Set the previous slide footer text to current's title
set prevSlide to mySlide - 1
set fText to "Coming up: " & content of text range of text frame of myTitle
set header footer text of footer of headers and footers of slide prevSlide of active presentation to fText
end if
set prevSlide to currentSlide
set mySlide to mySlide + 1
end repeat
set mySlide to mySlide - 1
set header footer text of footer of headers and footers of slide mySlide of active presentation to "End of presentation"
return currentSlide
end tell
To use this code open Script Editor.app
- Choose File->New
- Paste all the code in the window
- Save the file as: Documents/Microsoft User Data/PowerPoint Script Menu Items/addNextSlideFooter.scptd
- Open a PowerPoint presentation
- Ensure that footers are being shown (if they aren't just add a footer with any text in it. This script will REPLACE the footer text)
- Choose the automator menu (squiggly option to the right of Help)
- Choose the name of your script (addNextSlideFooter.scptd)
tell application "Microsoft PowerPoint" -- This just tells the script we're using PowerPoint
-- set X to Y just sets a variable. Similar to x = y in other languages.
set oPres to active presentation
set mySlide to 1
tell active presentation to set currentSlide to slide mySlide
-- We need to create a list of types that could possibly hold the title so we can get the title later.
-- Luckily, if you're using a PowerPoint template there are some specific title placeholders.
-- If you aren't using a template (or any of these placeholders) for the title, this whole script won't work.
set titleTypes to {placeholder type title placeholder, placeholder type center title placeholder, placeholder type vertical title placeholder}
set footerTypes to {placeholder type footer placeholder}
-- Loop over all slides in the presentation, setting currentSlide to the current slide in each loop.
repeat with currentSlide in (get every slide of oPres)
-- We set the title of the previous slide, so if we're on slide 1, skip it!
if mySlide > 1 then
-- Get the title of the current slide by searching all the shapes for one of
-- the correct type.
repeat with i from 1 to count shapes of currentSlide
set currentShape to shape i of currentSlide
set aType to placeholder type of currentShape
-- If there is a title, store it in the variable myTitle
if aType is not missing value and aType is in titleTypes then set myTitle to currentShape
end repeat
-- Get the footer of the previous slide (same this as the title, but for the previous slide)
repeat with i from 1 to count shapes of prevSlide
set currentShape to shape i of prevSlide
set aType to placeholder type of currentShape
if aType is in footerTypes then set myFooter to currentShape
end repeat
-- Set the previous slide footer text to current's title
set prevSlide to mySlide - 1
set fText to "Coming up: " & content of text range of text frame of myTitle
set header footer text of footer of headers and footers of slide prevSlide of active presentation to fText
end if
set prevSlide to currentSlide -- Update some variables
set mySlide to mySlide + 1
end repeat
-- Make sure the last slide says "End of presentation" in the footer.
set mySlide to mySlide - 1
set header footer text of footer of headers and footers of slide mySlide of active presentation to "End of presentation"
return currentSlide
end tell -- DONE!
No comments:
Post a Comment